MVVM 패턴에서는 Command 속성에 Binding을 해야만 클릭 시 ViewModel로 이벤트가 전달됩니다. Command 속성은 ICommand Type으로 ViewModel 역시 ICommand Type의 객체를 생성하여 Binding 합니다. Button을 예를 들어 코드를 작성하겠습니다.
✅ Command Binding
View
<Button Command="{Binding ButtonCommand}" Content="TEST" Width="100" Height="30"/>
버튼을 생성합니다. Command 속성에 ButtonCommand를 Binding 합니다.
ViewModel
private RelayCommand _buttonCommand;
public RelayCommand ButtonCommand
{
get
{
if (_buttonCommand == null)
_buttonCommand = new RelayCommand(OnButtonClicked);
return _buttonCommand;
}
}
private void OnButtonClicked()
{
// Code here
}
ButtonCommand는 RelayCommand Type이며 RelayCommand는 ICommand를 상속받아 구현되었습니다.
RelayCommand 가 필요하신 분은 다음 항목을 참고하시면 됩니다.
RelayCommand.cs
using System;
using System.Windows.Input;
namespace MVVM;
public sealed class RelayCommand : ICommand
{
readonly Action<object> _execute;
readonly Func<bool> _canExecute;
public event EventHandler CanExecuteChanged
{
add => CommandManager.RequerySuggested += value;
remove => CommandManager.RequerySuggested -= value;
}
public RelayCommand(Action execute) : this(execute, null)
{
// Delegated to
// RelayCommand(Action execute, Func<bool> canExecute)
}
public RelayCommand(Action<object> execute)
: this(execute, null)
{
// Delegated to
// RelayCommand(Action<object> execute, Func<bool> canExecute)
}
public RelayCommand(Action execute, Func<bool> canExecute)
{
if (execute == null)
throw new ArgumentNullException("execute");
_execute = p => execute();
_canExecute = canExecute;
}
public RelayCommand(Action<object> execute, Func<bool> canExecute)
{
_execute = execute ?? throw new ArgumentNullException("execute");
_canExecute = canExecute;
}
/// <inheritdoc cref="IRelayCommand.CanExecute" />
public bool CanExecute(object parameter)
{
return _canExecute?.Invoke() ?? true;
}
/// <inheritdoc cref="IRelayCommand.Execute" />
public void Execute(object parameter)
{
_execute(parameter);
}
}
RelayCommand는 웹에서 검색하면 많은 버전이 존재하니 편하신 걸로 사용하시면 되겠습니다. 대부분 비슷합니다. 제가 사용하는 버전을 다운로드할 수 있게 올려뒀습니다.
위와 같이 구현하면 최종적으로 버튼 클릭 시 OnButtonClicked 함수가 호출됩니다.
관련 포스팅
[WPF/xaml] - PasswordBox,TextBox: Enter 입력 시 Command 실행 (MVVM)
✅ Command Binding - 끝
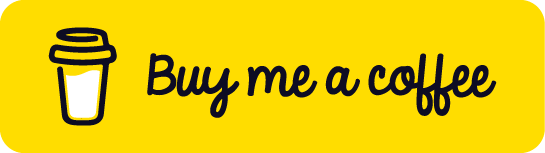