C#의 Console Application에서 Ctrl+C 를 입력했을 때 특별한 이벤트를 발생시키는 방법입니다. Console에서 구동 중인 Application을 중단할 때 종료 이벤트를 수행하는 것으로 로그 수집 종료, 메모리 반환 등의 코드가 수행되도록 처리하는 것이 일반적입니다.
✅ Console Application - Cancel (Ctrl+C)
ConsoleCancelEventHandler
using System;
class Program
{
static void Main(string[] args)
{
Console.CancelKeyPress += new ConsoleCancelEventHandler(Console_CancelKeyPress);
// Ctrl+C 가 발생할 때 까지 무한루프
while (true) { }
}
static void Console_CancelKeyPress(object sender, ConsoleCancelEventArgs e)
{
// 즉시 종료되는 것을 방지함
e.Cancel = true;
Console.WriteLine("Ctrl+C pressed");
}
}
람다 & delegate
람다, delegate를 사용하여 코드를 줄이고 전역 bool 을 사용하여 Ctrl+C 가 발생했을 때 무한루프를 종료하는 코드입니다.
https://stackoverflow.com/questions/177856/how-do-i-trap-ctrlc-sigint-in-a-c-sharp-console-app
How do I trap Ctrl+C (SIGINT) in a C# console app?
I would like to be able to trap Ctrl+C in a C# console application so that I can carry out some cleanups before exiting. What is the best way of doing this?
stackoverflow.com
class MainClass
{
private static bool keepRunning = true;
public static void Main(string[] args)
{
Console.CancelKeyPress += delegate(object? sender, ConsoleCancelEventArgs e) {
e.Cancel = true;
MainClass.keepRunning = false;
};
while (MainClass.keepRunning) {
// Do your work in here, in small chunks.
// If you literally just want to wait until Ctrl+C,
// not doing anything, see the answer using set-reset events.
}
Console.WriteLine("exited gracefully");
}
}
위 코드는 stackoverflow.com 에서 발췌했습니다.
✅ Console Application - Cancel (Ctrl+C) 끝
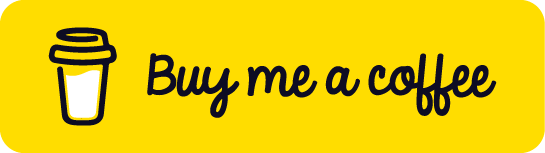
C#의 Console Application에서 Ctrl+C 를 입력했을 때 특별한 이벤트를 발생시키는 방법입니다. Console에서 구동 중인 Application을 중단할 때 종료 이벤트를 수행하는 것으로 로그 수집 종료, 메모리 반환 등의 코드가 수행되도록 처리하는 것이 일반적입니다.
✅ Console Application - Cancel (Ctrl+C)
ConsoleCancelEventHandler
using System;
class Program
{
static void Main(string[] args)
{
Console.CancelKeyPress += new ConsoleCancelEventHandler(Console_CancelKeyPress);
// Ctrl+C 가 발생할 때 까지 무한루프
while (true) { }
}
static void Console_CancelKeyPress(object sender, ConsoleCancelEventArgs e)
{
// 즉시 종료되는 것을 방지함
e.Cancel = true;
Console.WriteLine("Ctrl+C pressed");
}
}
람다 & delegate
람다, delegate를 사용하여 코드를 줄이고 전역 bool 을 사용하여 Ctrl+C 가 발생했을 때 무한루프를 종료하는 코드입니다.
https://stackoverflow.com/questions/177856/how-do-i-trap-ctrlc-sigint-in-a-c-sharp-console-app
How do I trap Ctrl+C (SIGINT) in a C# console app?
I would like to be able to trap Ctrl+C in a C# console application so that I can carry out some cleanups before exiting. What is the best way of doing this?
stackoverflow.com
class MainClass
{
private static bool keepRunning = true;
public static void Main(string[] args)
{
Console.CancelKeyPress += delegate(object? sender, ConsoleCancelEventArgs e) {
e.Cancel = true;
MainClass.keepRunning = false;
};
while (MainClass.keepRunning) {
// Do your work in here, in small chunks.
// If you literally just want to wait until Ctrl+C,
// not doing anything, see the answer using set-reset events.
}
Console.WriteLine("exited gracefully");
}
}
위 코드는 stackoverflow.com 에서 발췌했습니다.